You want to add form validation. But as a flutter beginner, you need a step-by-step guide to add form validation.
For complete understanding, you will create a form and then you validate the form.
I recommend don’t just read this tutorial guide. Also, create a sample project to follow along the journey.
You have to follow these five steps.
In this Article
Step 1 – Create a Form Widget
Go to the Scaffold()
widget (which represents screens in Flutter).
The scaffold has a body parameter.
Pass Form widget in the body of the scaffold.
The Form()
widget that you created has key and child parameters.
Create a GlobalKey
You create a GlobalKey
of generic type FormState
and assign it to a variable name formKey
.
final formKey = GlobalKey<FormState>();
Now, pass this formKey variable to the key property of the Form()
widget.
body: Form(
key: formKey,
child:
),
This code snippet will produce some problems because our child parameter is empty.
Create a Column Widget
Assign a Column()
widget to the child property of the Form()
widget.
Column()
has a children parameter.
body: Form(
key: formKey,
child: Column(
children: [],
),
),
children take the list of Widgets and show it to the UI as a vertical list of UI.
Step 2 – Create TextFormField Widgets
Inside the children
list, you will create two TextFormField
widgets.
Note: You have to create TextFormField
(Not TextField
).
body: Form(
key: formKey,
child: Column(
children: [
TextFormField(),
TextFormField(),
],
),
),
Perfect!
What You Will Create
Here, you will take the username and password for login (Just example project).
You validate the username and password fields. And make sure that they (username and password fields) are not empty.
Let’s Create Hint
A hint is useful for end-users. So they can see what they can write in these text fields.
TextFormField
has many parameters.
But here you will use decoration and obscureText for the moment.
decoration: You pass InputDecoration()
.
obscureText: You pass true (Only for the password field).
body: Form(
key: formKey,
child: Column(
children: [
TextFormField(
decoration: const InputDecoration(hintText: 'Username'),
),
TextFormField(
decoration: const InputDecoration(hintText: 'Password'),
obscureText: true,
),
],
),
),
You pass hintText to the InputDecoration
.
Step 3 – Create an ElevatedButton Widget
You need a Login button when the user press that button then you will validate the user inputs.
ElevatedButton(onPressed: (){}, child: const Text('Login'))
Now, let’s just pass this elevated button to the column that we have created inside the Form()
widget before.
body: Form(
key: formKey,
child: Column(
children: [
TextFormField(
decoration: const InputDecoration(hintText: 'Username'),
),
TextFormField(
decoration: const InputDecoration(hintText: 'Password'),
obscureText: true,
),
ElevatedButton(onPressed: (){}, child: const Text('Login'))
],
),
),
Step 4 – Add Validator Property
TextFormField
has a validator parameter.
The validator
will take an anonymous method.
validator: (value) {
if (value == null || value == '') {
return 'Enter your username.';
}
return null;
},
Inside the anonymous function, you will receive an argument String by the framework.
You can perform any check on this validator function.
Here, we are checking if the value (User TextField input) is null or value is empty. Then we return the String “Enter your username.”.
This message will show on the UI if the user forgets to write in the TextField
.
Now Let’s see the TextField
code snippet
TextFormField(
decoration: const InputDecoration(hintText: 'Username'),
validator: (value) {
if (value == null || value == '') {
return 'Enter your username.';
}
return null;
},
),
TextFormField(
decoration: const InputDecoration(hintText: 'Password'),
obscureText: true,
validator: (value) {
if (value == null || value == '') {
return 'Enter your password.';
}
return null;
},
),
Step 5 – Validate Form in the onPressed
This is the MOST IMPORTANT part because here you will trigger this validator method.
ElevatedButton(
onPressed: () {
if (formKey.currentState!.validate()) {
//This is the actual action
}
},
child: const Text('Login'),
)
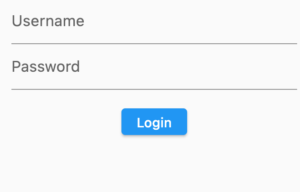
This elevated button will execute the validator methods that we pass to the above text Fields.
onPressed parameter of ElevatedButton will take a method. And in this method, we are checking if formKey (which is the Global Key that we declare before) currentState is validated then we execute the actual action code inside the check.
Look at The Complete Example
import 'package:flutter/material.dart';
class FormValidation extends StatelessWidget {
const FormValidation({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
final formKey = GlobalKey<FormState>();
return Scaffold(
body: Form(
key: formKey,
child: Center(
child: SizedBox(
width: 300,
child: Column(
children: [
TextFormField(
decoration: const InputDecoration(hintText: 'Username'),
validator: (value) {
if (value == null || value == '') {
return 'Enter your username.';
}
return null;
},
),
TextFormField(
decoration: const InputDecoration(hintText: 'Password'),
obscureText: true,
validator: (value) {
if (value == null || value == '') {
return 'Enter your password.';
}
return null;
},
),
const SizedBox(height: 20),
ElevatedButton(
onPressed: () {
if (formKey.currentState!.validate()) {}
},
child: const Text('Login'),
)
],
),
),
),
),
);
}
}

Now you can load this Widget Screen to see the preview.
Conclusion
Hope you just understand the basics of validation in Flutter.
Thanks!